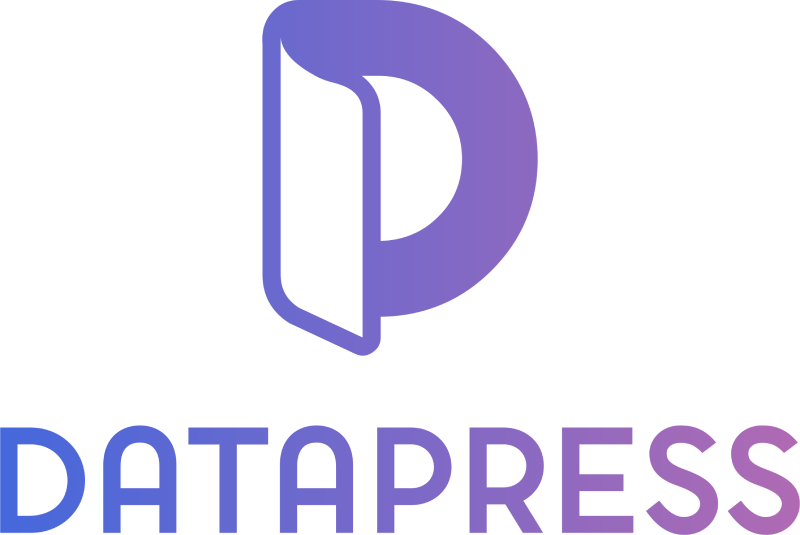
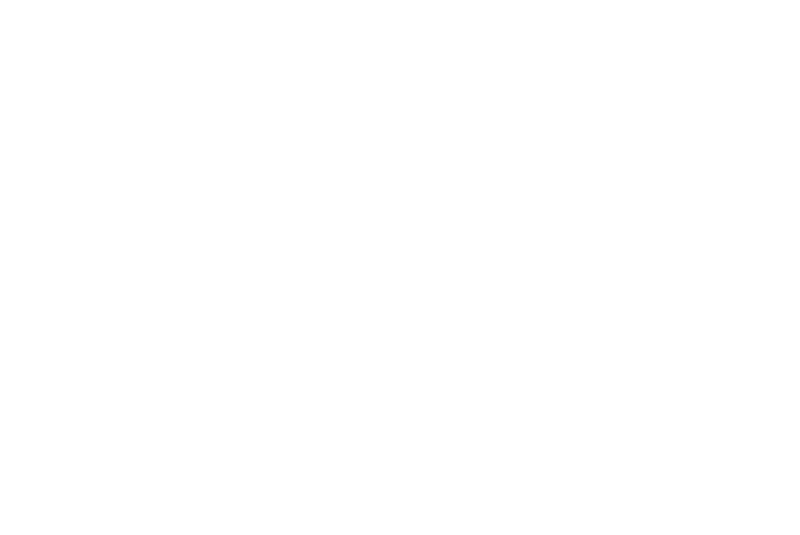
API Reference
Uploading Files
Version:Â 4.0
Updated:Â 14th July 2024
Updated:Â 14th July 2024
Adding a file
The file upload API uses multipart/form-data
with a file
attachment.
- The
dataset.updatedAt
field will automatically be updated to the current time. - Other metadata fields can be edited through the PATCH API.
POST
https://data.website/api/dataset/:id/resourcesPath Parameters
:id | 5-character ID of the dataset, eg. "ab12x" | string | Required |
HTTP Headers
Authorization | API key with editor permissions. | string | Required |
Content-Type | Must be "multipart/form-data". Most clients will set this automatically. | string | Required |
Form Fields
file | The file to upload. | file | Required |
title | The title of the resource on the page. | string | Optional |
HTTP Return Codes
200 | Returns the full dataset JSON. |
400 | Returns a JSON object explaining the error. |
403 | API key does not have editor permissions. |
Example: Upload a CSV file
The newly uploaded CSV is added to the top of the file list on the webpage.
import requests, os, json
dataset_id = "20abc"
url = f"https://data.website/api/dataset/{dataset_id}/resources"
headers = {
"Authorization": os.getenv("DATAPRESS_API_KEY")
}
# Prepare the file and metadata
with open('example.csv', 'rb') as file:
files = {
'file': ('example.csv', file, 'text/csv')
}
# Optional metadata field
data = { 'title': 'Monthly Spending Data' }
# Requests will set Content-Type automatically
response = requests.post(url, files=files, data=data, headers=headers)
print(json.dumps(response.json(), indent=2))
{
"id": "20abc",
// [...dataset JSON]
"updatedAt": "...", // Updates to the current time
"resources": {
"0ck": {
"title": "Monthly Spending Data",
"description": ""
"timeFrame": null,
"order": 0, // The file is added to the top of the list
// Immutable fields:
"origin": "site/dataset/20abc/.../example.csv", // Auto-assigned
"format": "csv", // Auto-detected
"hash": "abc123456", // MD5 hash of the file
"size": 1024, // Auto-detected, bytes
},
},
}
Replacing a file
To replace an existing file, use the same multipart/form-data
format but include the resource ID in the URL path.
- The
dataset.updatedAt
field will automatically be updated to the current time. - Other metadata fields can be edited through the PATCH API.
POST
https://data.website/api/dataset/:id/resources/:resource_idPath Parameters
:id | 5-character ID of the dataset, eg. "ab12x" | string | Required |
:resource_id | 3-character ID of the resource to replace, eg. "0ck" | string | Required |
HTTP Headers
Authorization | API key with editor permissions. | string | Required |
Content-Type | Must be "multipart/form-data". Most clients will set this automatically. | string | Required |
Form Fields
file | The new file to upload. | file | Required |
title | The title of the resource on the page. | string | Optional |
HTTP Return Codes
200 | Returns the full dataset JSON. |
400 | Returns a JSON object explaining the error. |
403 | API key does not have editor permissions. |
404 | The dataset or resource does not exist. |
Example: Replace a CSV file
This overwrites the existing resource file and updates the title.
import requests, os, json
dataset_id = "20abc"
resource_id = "0ck"
url = f"https://data.website/api/dataset/{dataset_id}/resources/{resource_id}"
headers = {
"Authorization": os.getenv("DATAPRESS_API_KEY")
}
# Prepare the replacement file
with open('updated.csv', 'rb') as file:
files = {
'file': ('updated.csv', file, 'text/csv')
}
# Optional metadata field
data = { 'title': 'Monthly Spending Data (Updated)' }
# Requests will set Content-Type automatically
response = requests.post(url, files=files, data=data, headers=headers)
print(json.dumps(response.json(), indent=2))
{
"id": "20abc",
// [...dataset JSON]
"updatedAt": "...", // Updates to the current time
"resources": {
"0ck": {
"title": "Monthly Spending Data (Updated)",
"description": ""
"timeFrame": null,
"order": 0, // Position in list is preserved
// Updated immutable fields:
"origin": "site/dataset/20abc/.../updated.csv",
"format": "csv",
"hash": "def789012",
"size": 1536,
},
},
}