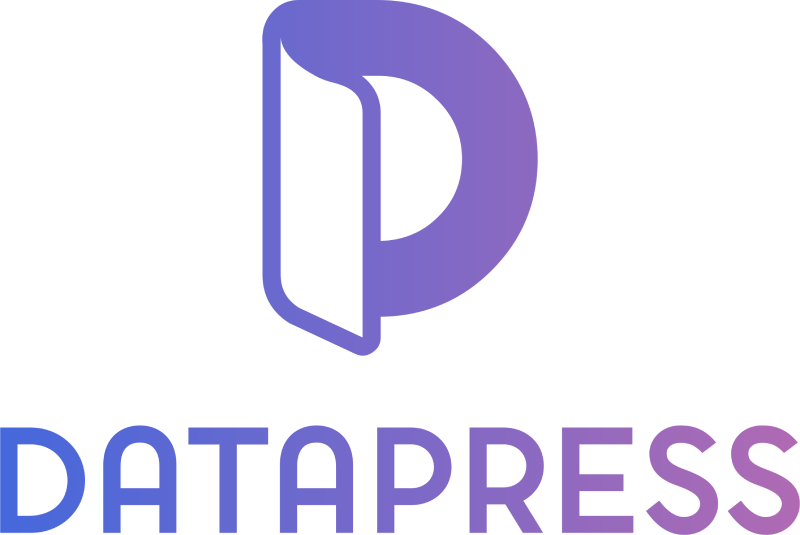
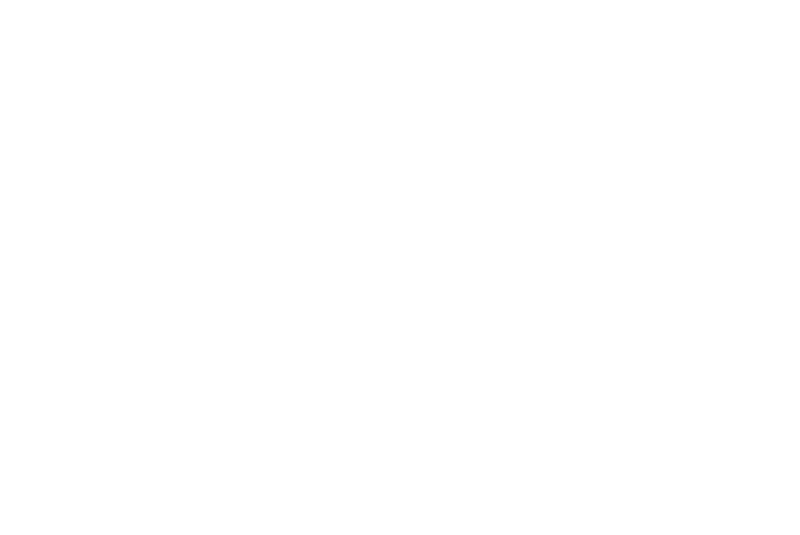
API Reference
Teams
Version:Â 4.0
Updated:Â 14th July 2024
Updated:Â 14th July 2024
Teams are groups of users who can collaborate on datasets. Every dataset is owned by a team.
- They are indexed using a 5-character ID, eg.
ab12x
. - Users are stored as numeric IDs.
List all teams
GET
https://data.website/api/teamHTTP Return Codes
200 | Returns a JSON list of teams. |
/api/team
{
"05jg5": {
"title": "Housing Team",
"users": [ 10, 62, 431 ],
"site": "mysite", // Immutable
"id": "05jg5" // Immutable
},
"59m9r": {
"title": "Health Intelligence",
"users": [ 384, 24, 1049 ],
"site": "mysite", // Immutable
"id": "59m9r" // Immutable
},
// ...
}
Update a team
Accepts a JSON Patch request body.
PATCH
https://data.website/api/team/:idPath Parameters
:id | 5-character ID of the team, eg. "ab12x" | string | Required |
HTTP Headers
Authorization | API key with editor permissions. Must be an admin or a team member. | string | Required |
Content-Type | Must be "application/json". Most clients will set this automatically. | string | Required |
HTTP Return Codes
200 | Returns the updated team. |
Example: Removing a user
To remove user 1049
from a team:
import requests, os, json
headers = { "Authorization": os.getenv("DATAPRESS_API_KEY") }
patch = [
{
# We use a JSON PATCH test operation to verify
# that we are removing the correct user.
"op": "test",
# The offset of the user in the list
"path": "/users/2",
# The ID of the user to remove
"value": 1049
},
{
# This will only run if the test operation succeeds.
"op": "remove",
"path": "/users/2"
}
]
url = f"https://data.website/api/team/59m9r"
# This will submit the patch with the header "Content-Type: application/json"
response = requests.patch(url, json=patch, headers=headers)
print(json.dumps(response.json(), indent=2))
{
"title": "Health Intelligence",
// This has been updated:
"users": [ 384, 24 ],
"site": "mysite", // Immutable
"id": "59m9r" // Immutable
}
Create a team
POST a partial JSON object to create a new team. Valid fields are:
title
, requiredusers
, optional
POST
https://data.website/api/teamHTTP Headers
Authorization | API key with editor permissions. Must be an admin. | string | Required |
Content-Type | Must be `application/json`. Most clients will set this automatically. | string | Required |
Example: New team
import requests, os, json
headers = { "Authorization": os.getenv("DATAPRESS_API_KEY") }
team = { "title": "New Team" }
url = f"https://data.website/api/team"
response = requests.post(url, json=team, headers=headers)
print(json.dumps(response.json(), indent=2))
{
"title": "New Team",
"users": [],
"site": "mysite", // Immutable, auto-assigned
"id": "tm123" // Immutable, auto-assigned
}
Delete a team
To be eligible for deletion, the team must not be the owner of any datasets.
Delete all the team's datasets first, or assign them to a different team.
DELETE
https://data.website/api/team/:idPath Parameters
:id | 5-character ID of the team, eg. "ab12x" | string | Required |
HTTP Headers
Authorization | API key with editor permissions. Must be an admin. | string | Required |
HTTP Return Codes
200 | Team deleted. |
404 | Team not found. |
403 | Not an admin. |
400 | Team still has datasets. |